Web Client Application
Bootstrap the frontend application
In this section, you will use Angular 8 to create the frontend application project.
If you do not have Angular CLI installed on your machine, open a terminal window and run npm install -g @angular/cli@8
. This will install the version 8 of Angular.
To create the frontend application
- In a terminal, navigate to the same root directory as the backend application directory.
- Run
ng new events-ui
and accept the defaults. This creates the frontend project directory named events-ui and installs Angular8 dependencies. - Open the project directory in your favorite frontend IDE.
- Create or edit the following folders and files in the project directory:
- /src/app/model directory - to create
- /src/environments directory - to edit
- /src/app/component directory - to create
- /src/app/app.module.ts file - to edit
Model
To implement the model
- Create a directory named model
- In the model directory create the event.ts file which defines the event received on the web-socket.
- Write the following code for the event class:
model/event.ts
Environment
The environments directory is created by default at the ng new
command. It contains 2 files:
- environment.ts - the default configuration file with the default environment settings
- environment.prod.ts - the production specific configuration file
To edit the environment.ts
file
- Open environment.ts and copy the following code in it:
environments/environment.ts
- Replace
<%WEBSOCKET-URL%>
with the public web socket endpoint of the events-application server app. For examplehttp://localhost:8080/socket
, if the app is running on localhost.
Component
To implement the component
directory
- Create a component directory named component
- By default, Angular8 creates component files. Their names start with app.component. Move the following types of component files to the previously created directory:
- app.component.ts - which stores the service that initiates web-socket and receives events on it
- app.component.html - which stores the display of the component
- app.component.css - which stores the style for the component
- Write the following code for the app.component.ts:
component/app.component.ts
- Import the required packages for app.component.ts above the class declaration.
component/app.component.ts
- Write the following code for the app.component.html:
component/app.component.html
- Write the following code for the app.component.css:
component/app.component.css
Application Module
The module file stores the links to other modules and the imports for the frontend application.
To edit the app.module.ts
file
- Open the typescript file named app.module.ts
- Write the following code for the app.module.ts
app.module.ts
- Import all the required libraries and modules:
app.module.ts
Edit Other Angular Default Files
For the frontend application to work, you need to edit some of the default angular files, created by the ng new name-of-the-app
command.
Find the files that need editing in the src/ directory:
- main.ts - the main entry point for your app. Compiles the application with the JIT compiler and bootstraps the application’s root module AppModule to run in the browser.
main.ts
- polyfills.ts - the polyfill scripts that make the application compatible with different browsers
polyfills.ts
- styles.css - the global style file
styles.css
- index.html - the main HTML page that is served when you access the default port.
index.html
- angular.json - CLI configuration defaults for all projects in the workspace, including configuration options for build, serve, and test tools that the CLI uses
angular.json
This UI application was built with Angular 8.2.9 version. Using a greater version may lead to conflicts for the imports of dependencies and modules. To resolve the conflicts, modify the package.json file as follows and run
npm install
after saving the file.
- package.json - the npm package dependencies that are available to all projects in the workspace
package.json
This is how your frontend project should look like at the end:
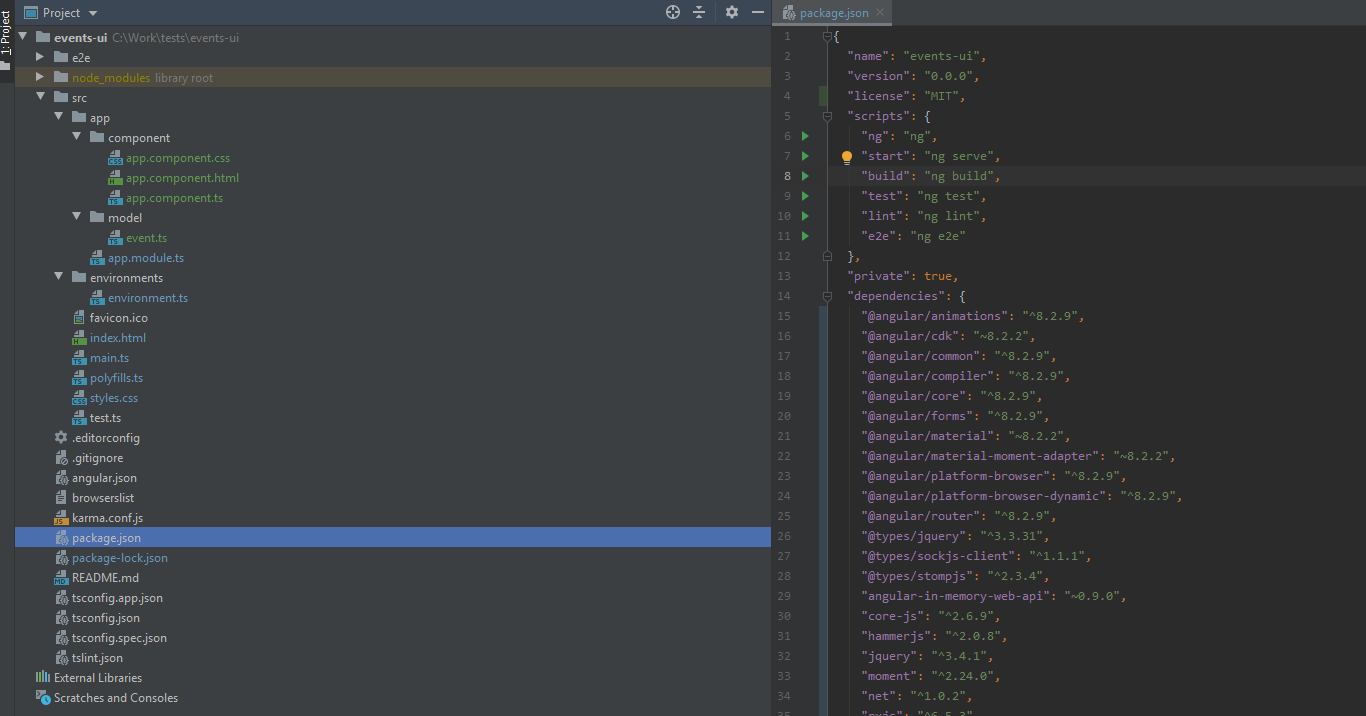
Events App Frontend Project