Backend Application
Bootstrap App
In this section, you will use a web service called Spring Initializr to prepare the Spring Boot project.
To bootstrap your application
- Go to https://start.spring.io/ to use Spring Initializr.
- Enter the Project Metadata, as follows:
- Group: the group ID, such as
com.finastra
- Artifact: the artifact ID, which is your application name, such as:
events
- Group: the group ID, such as
- Click Generate. A ZIP archive, with the name of your artifact, is ready for you to download.
- Unpack the downloaded archive, and open the project in your favorite IDE.
- Open pom.xml. The
<parent>
version must be the same as the version ofspring-boot-...
dependencies. To do this automatically, add thespring-boot.version
property as a variable in the<properties>
field from the pom.xml file.
pom.xml
- In pom.xml add a set of
<dependencies>
from Maven repository , apart from the Spring Initializr ones. Use the${spring-boot.version}
value for the spring-boot dependencies<version>
key.
pom.xml
- Update the
<plugins>
element as follows:
pom.xml
- Create a configuration file, named openapi.yaml, in /src/main/resources/. Enter the following content:
openapi.yaml
This file is the YAML representation of the Events specification in OpenAPI format.You can find it under Actions menu. Make sure to convert it to YAML format for your project.
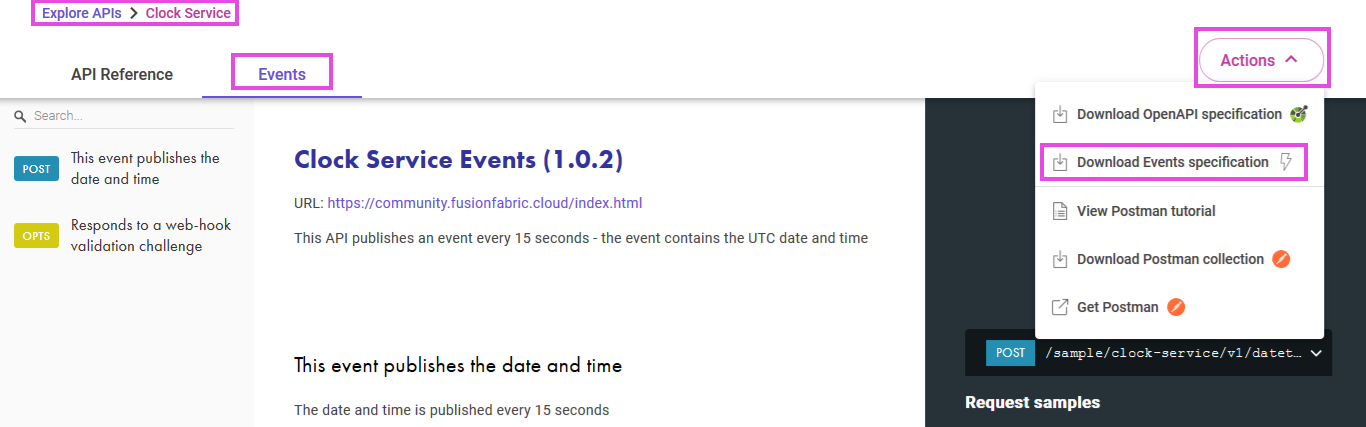
API Swagger files
You are now ready to code your event notifications application.
By setting up webhooks in your backend application you will receive notifications from FusionFabric.cloud any time the event occurs.
You will be using a code generator Maven plugin - swagger-codegen-maven-plugin
from io.swagger
, to help you implement the two endpoints of the Clock Service Events specification, namely:
POST /sample/clock-service/v1/datetime-published
- to return the date and time at every 15 seconds.OPTIONS /sample/clock-service/v1
- to allow FusionFabric.cloud to test your webhook URLs. This endpoint will return an HTTP header -WebHook-Allowed-Origin
with the valueapi.fusionfabric.cloud
.
You create 4 packages in the src/main/java/com.finastra.events/ directory:
- controller package - which stores a REST controller object
- configuration package - which stores configuration objects
- exceptions package - which stores exception objects
- filter package - which stores filtering objects applied to any incoming HTTP requests
Controller
To implement the controller
package
- Create a package named controller.
- In Controller package, create the EventsApplicationController class with the following code:
controller/EventsApplicationController.java
- Import the required libraries for EventsApplicationController above the class declaration.
controller/EventsApplicationController.java
Configuration
To implement the configuration
package
- Create a package named configuration.
- In the configuration package, create 2 classes:
- MessagingConfiguration
- WebSocketConfiguration
- Write the following code for the MessagingConfiguration class:
configuration/MessagingConfiguration.java
- Import the required libraries for MessagingConfiguration above the class declaration.
configuration/MessagingConfiguration.java
- Write the following code for the WebSocketConfiguration class:
configuration/WebSocketConfiguration.java
- Import the required libraries for the WebSocketConfiguration above the class declaration.
configuration/WebSocketConfiguration.java
Exceptions
To implement the exceptions
package
- Create a package named exceptions.
- In exceptions package, create the JwksException class with the following code:
exceptions/JwksException.java
Resource Sharing Filter
To implement the filter
package
- Create a package named filter.
- In the filter package, create 4 classes:
- CorsFilter
- MutipleReadHttpRequest
- SignatureCheckFilter
- TraceFilter
- Write the following code for the CorsFilter class:
filter/CorsFilter.java
- Import the required libraries for CorsFilter above the class declaration.
filter/CorsFilter.java
- Write the following code for the MultipleReadHttpRequest class:
filter/MultipleReadHttpRequest.java
- Import the required libraries for MultipleReadHttpRequest above the class declaration.
filter/MultipleReadHttpRequest.java
- Write the following code for the SignatureCheckFilter class:
filter/SignatureCheckFilter.java
- Import the required libraries for SignatureCheckFilter above the class declaration.
filter/SignatureCheckFilter.java
- Write the following code for the TraceFilter class:
filter/TraceFilter.java
- Import the required libraries for TraceFilter above the class declaration.
filter/TraceFilter.java
Application Properties
To configure the application properties
- In /src/main/resources open the application.properties file, created by default
- Add the following content:
application.properties
This is how your backend project should look like at the end:
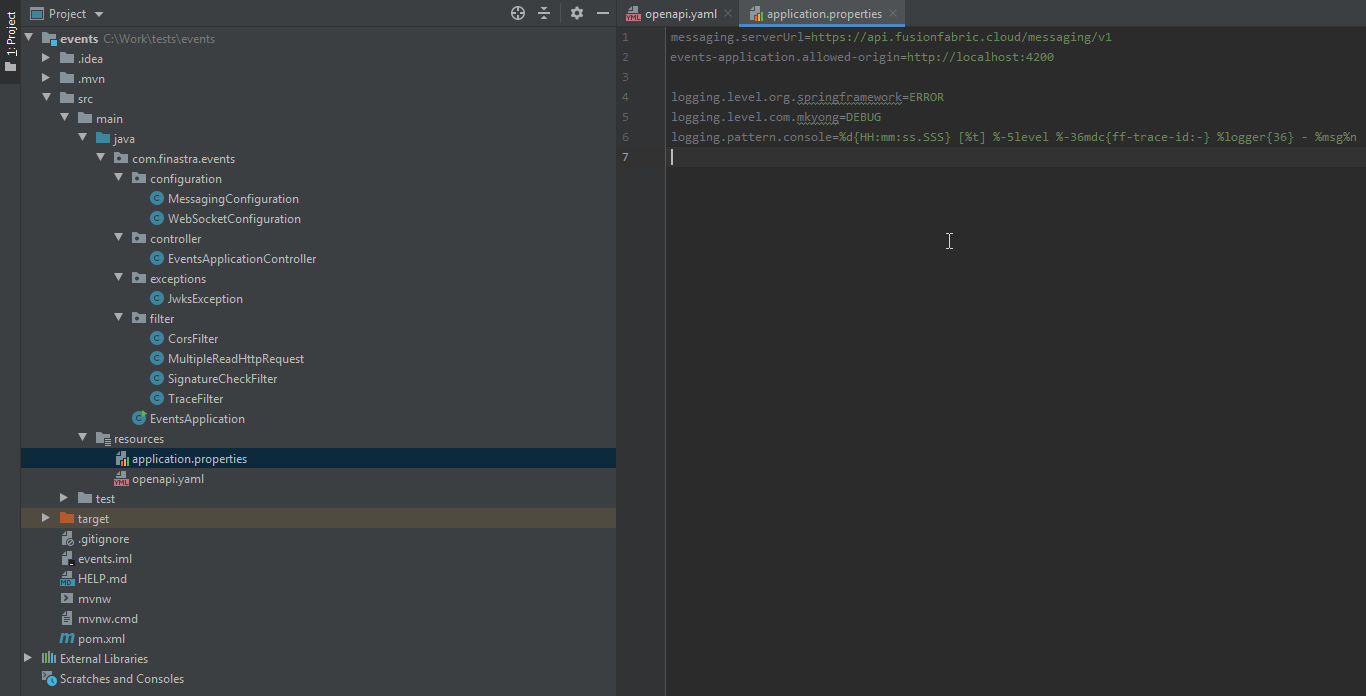
Events App Backend Project